We need a tutorial on how to create a font tester in HTML / CSS / JS
Options
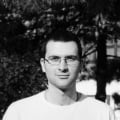
Igor Petrovic
Posts: 325
Just an idea, I think it would be great if some of the code-savvy folks here would be willing to make a tutorial on this. As I see it, it's the main component that is highly specific to font sites, and I guess it would make life easier for independent type designers and foundries.
Or is there already one that I overlooked?
Or is there already one that I overlooked?
-1
Comments
-
I worked on a HTML/CSS/JS implementation of Galvanized Jets for Samarskaya and Partners. If you want to know how it's done, the code is all available on GitHub. That may be worth a read because it's a clean design (TypeScript) which doesn't use any of the big JS page design frameworks (React/Vue/whatever is in this year).
I'm not going to write a complete tutorial because although it is quite specific to font sites, like all programming it's just a matter of thinking through the different steps of what you need to get done and how you're going to do it, and much of that is specific to the kind of site you're making.
Do you mean "font tester" from the point of view of the type designer or the potential purchaser? The answer's going to be different, but there'll be some overlap. Assuming the first:- You need a way for the user to drop/upload the font onto the web site, and for you to then display text in it. I chose to use fontdrag.js from Ryan Seddon in Galvanized Jets, because that takes care of the main steps: decoding the font from the File object into a data: URI object; adding the URI to a CSS font-face rule; calling a callback to register the font with the rest of your testing app. But in other cases (like Crowbar and Fontpainter) I've done this by hand, using React Dropzone components as a base.
- You need to be able to inspect the font and see what it can do (variable axes, glyphs available, etc). I've done this in different ways in the past; Galvanized Jets used a variable-aware fork of opentype.js, but these days I'd probably use fontkit. If I were doing very custom stuff I might use harfbuzzjs.
- You need to provide an interface to variation sliders, and alter the CSS properties of your text based on user input. varfont.ts in Galvanized Jets is a good example of how to do that.
If you have any more specific questions I'd be happy to answer.4 -
Thanks Simon! This sheds light on the general approach behind "live font" behavior on the web. Going further into the discussion, I was thinking about simplier case — that is to enable customer who visits type foundry's site to try some text, change text size, check OT features and see variable axes.
The idea is to help independent type designers to have their website (which I find very important given the situation on the market). For those of us who don't code it might be a big pain point that leaves us to the mercy of ~Corporations~ or direct us to the (in my opinion) expensive subsription solutions.
For example, at the moment I am able to do full HTML/CSS, but still don't know JS part. I am not sure I am in situation to dive into learning JS, but it would be really helpful for me to see a tutorial with JS part executed and commented (explaining the purpose of each step). Then I could have a general overview and insert the "JS module" into my existing site (and maybe even tweak JS here and there).
Nowadays there are solutions on the web to make your own website relatively easily, but still not for selling fonts. If we could manage to have this minimal type tester (for customer) as a clean copyable HTML/CSS/JS module that might be a bridge to those other "realtively easy" solutions.0 -
Hi Igor. I suspect you would be able to license a type tester from among the various ones that already exist. The one we’re using for our upcoming new website was written by Kenneth Ormandy, and he provided it along with the other back-end work we hired him to do.2
-
1
-
Thanks Enrico, I'll take a closer look, but at first glance, it looks super-helpful and very close to what I had in mind!
Thanks John, I was hoping that it would be in the form of a code snippet like many HTML/CSS/JS tutorials on the web. Does it still need a license?
0 -
Hi Igor. If you’re asking for a tutorial, it sounds like you want someone to explain what each part of a font tester does. Here’s a minimal live font tester as an example to build from. Copy this code and save it as an HTML file, then open it in your browser.
<html> <section class="try-me" contenteditable>Try me</section> <style class="my-css-rules" contenteditable>.my-css-rules { display: block; } .try-me { font-size: 150px; }</style> </html>
This example works by exposing the CSS that styles the page. A style element is hidden by default, but you can unhide it by applying a display: block rule to it.
Want to change the font? Specify the font-family.
Want to change it to one of your fonts? Use the @font-face rule to tell it where your font is.
Want to clean up your code so that the only CSS you see is what applies to the font tester? Add another style element at the top and move the other CSS rules there.
Later as you add Javascript to the page to listen for things like sliders or drop-down menus changing, you will learn how to automate the process of adding, changing, and removing CSS rules.2 -
Thanks David, this is also a super helpful piece of information for me! I'll try and experiment with it.
EDIT: Now I understand the principle, this is the perfect level of complexity/simplicity. If one knows where to find more resources like this short example, I would appreciate it0 -
Very happy to hear it helped, Igor. Got another one for you.
<html> <section id="demo" class="try-me" style="font-size: 100px;" contenteditable>Try me</section> <input type="range" name="size" id="slider" min="10" max="200" value="100" step="1"> </html>
We’ll start with another simple HTML structure. Note that instead of using a style element like last time I’m using an inline style style="font-size: 100px;" since it’s a little easier to understand what’s happening when we change it dynamically. Then we’ll add a script tag with some Javascript to connect these two elements.<html> <section id="demo" class="try-me" style="font-size: 100px;" contenteditable>Try me</section> <input type="range" name="size" id="slider" min="10" max="200" value="100" step="1"> <script> var demo = document.getElementById("demo"); var slider = document.getElementById("slider"); slider.addEventListener("input", function() { demo.style.fontSize = slider.value + "px"});</script> </html>
After declaring our two variables, one representing the “Try me” demo element, and the other representing the slider, we now have a way of referring to each of these in our code.
In line 3 we add something called an event listener to the slider so our code can respond whenever the slider receives an "input" event (whenever someone’s dragging around our slider). Try changing "input" to "change" to see the difference between these two kinds of events.
Line 4 of our Javascript is the function that is executed each time our event listener “hears something.” It says the demo element’s inline style should be replaced by a new value (the slider’s value). The + "px" is concatenated; this means we’re literally tacking on the px unit to the end of the value. For example if the value of the slider is 144, then the new inline style for the demo element will be font-size: 144px;1 -
Hi @Igor Petrovic !I made one (html+css+js) for my inner purposes. Didn't plan to make it public because there are already many such tools out there. But if you like it, I can send a copy to you, privately.I attached the video with a quick demo.Here you can change/turning on/off:
– Font size (slider) – you can add notches, for example, on 16, 24, 48 pt, or wherever you want.
– Leading (slider).
– Tracking (slider).
– Variable font axes (sliders) – very easy to add new axes with their ranges.
– Dark mode inversion.
– Font family styles choosing (drop down menu).
– Text snippets (I use it for testing different languages, figures, kerning, so on) – you can add your own easily.
– OpenType Features, Stylistic Sets, Kerning and so on (buttons for turning on/off) – most of them are already there, but very easy to add new features.4 -
The actual "type testing" is as trivial as using webfonts; it's the UI that everybody has different ideas about, and how to integrate it in a foundry site.
2 -
@Igor Petrovic You can check fontsampler-js and see if you can make it work for your site; include the files, define the config and load some webfonts
3 -
If you want a tool that spits out bare-bones HTML, CSS and JavaScript to get your started, take a look at Specimen Skeleton. It sets up a type tester for your (variable) font, and a character grid. It's meant to give you the absolute minimum to build your own specimen site from.
The produced code is terse as possible, while still taking into account some of the browser quirks and bugs, which would be a great starting point to learn how the JavaScript, font and HTML interact. (Alternatively, you can take a look at the main JavaScript that has all the good bits).
3 -
One of the BIG “variables” in a type tester is whether it supports variable fonts
And how well
And that vs, or as well as, big families3 -
These are all awesome resources, many thanks to all for your recommendations and insights! Now it's time to digest and experiment0
-
I learned from @Michael Rafailyk about how frontend rendering (CSS/JS) exposes the web font files and could be the leak point in the font business model. And that the alternative way is backend rendering (browser text input goes to server > server renders string as an image > server sends the image back to the browser).
However, if my customers later use font files on their websites, that's also the leak point, right? But that also makes thieves work harder, to chase fonts in the wild instead of harvest all the library in one place.
I got a rough estimation that making the described backend rendering could be a day of work or so for the PHP guy.
What are your thoughts on all of this?
0 -
For me the best compromise is still a limited character set web font. Obviously the danger is there but it's also so much more reactive for testing. I also tried to mask my web fonts as much as I could but I have no idea if a decently skilled person* can find them in no time and I have a hard time believing that I can deceive an evil spider-bot anyway. Maybe some precautions and a fair selling price is the best answer.PS: Trial fonts are worrisome to me, but I understand how useful they can be.* let me know1
-
Enrico Sogari said:* let me know
the advice I've seen on typedrawers from people who sell a lot of fonts is that anti-piracy measures aren't worth the trouble; even if you lock down your own website, the retail fonts will make their way to shady forums, etc.
with that said there is more you can do on your own site, if you want (set a cross-origin policy, split the fonts into multiple files and use them in a CSS fallback chain, base64 encode them, etc)2 -
People who buy your fonts might use pirated fonts before buying them—conversely, someone pirating your fonts who was never going to pay for your fonts is also never going to buy them, anyway. All these measures that make paying customers’ lives worse are neither worth their time or cost, nor are they a good investment of your own energy.9
-
Thank you @jeremy tribby - I agree with that, it's fun to make it harder though. I made some changes, hopefully only the woff files that I use for the name of the fonts are easy to find but not the ones that I use for testing. If that was the case I could drastically reduce the former character-set to the font name letters. With all that said, yes there's no way to protect a file that ppl regularly buy.
1 -
Enrico Sogari said:I could drastically reduce the former character-set to the font name letters.
Regarding the frontend rendering, I know the platforms and foundries that provide the font testing using direct browser rendering, and it works well for them. In the same time, the backend rendering is not so difficult to implement using some PHP library, but the rendering time is a bit longer and the people will experience some delay when typing.2 -
If it is of any help, as a graphic designer that licenses a handful of fonts personally every year, and primarily works in branding—thus helping determine which fonts are licensed/used in projects—I won’t even consider a font from a foundry that does not include live font files on their site.
Seeing how the fonts actually render on screen is extremely important to my work. A png, jpg, or even SVG rendering does not suffice in my opinion.
My philosophy is, if you are too scared to host your own fonts, then why the hell do you even sell web fonts?
7 -
@Matthew Smith Does the backend rendering described above that delivers back PNG to the frontend (like MyFonts, Creative Market, and other big players do) also fall into a not acceptable method for you?
Basically, you prefer a raw frontend font rendering so you can evaluate the result?
0
Categories
- All Categories
- 46 Introductions
- 3.8K Typeface Design
- 478 Type Design Critiques
- 556 Type Design Software
- 1.1K Type Design Technique & Theory
- 644 Type Business
- 833 Font Technology
- 29 Punchcutting
- 510 Typography
- 120 Type Education
- 315 Type History
- 75 Type Resources
- 109 Lettering and Calligraphy
- 30 Lettering Critiques
- 79 Lettering Technique & Theory
- 534 Announcements
- 86 Events
- 110 Job Postings
- 167 Type Releases
- 170 Miscellaneous News
- 274 About TypeDrawers
- 53 TypeDrawers Announcements
- 119 Suggestions and Bug Reports